Rest API and Python API Documentation
In the context of this documentation, “Valispace“ will be called “Requirements and Systems Portal“.
This document provides the reference for the Requirements and Systems Portal's REST API. Using the REST API, the users can get and modify data from the Requirements and Systems Portal or even write data back into the Requirements and Systems Portal and thus update information. Additionally, this gives you the possibility to connect/integrate with other applications or apps.
Accessing the Rest API in the Requirements & System Portal (RSP) using the tokens
To access the REST API in the RSP app within Altium A365, the users can generate the tokens by navigating to the Settings menu and selecting User Tokens. Within the User Tokens settings page, you will find the API address for your specific deployment. This address is necessary for making API calls.
Click the “+” icon on the User Tokens page to generate a new token. Each token is valid for 3 months and must be regenerated after it expires.
Consuming the API using the access_token, adding a token through a "Bearer ..." using the application type "JSON" to the Authorization HTTP request header is necessary.
curl --location 'http://deployment_name/rest/requirements/versions/search/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer hO2lwhLZsYOgXPNVI' \
--data '{
"size": 10,
"query_filters": {
"object_id": 93
}
}'
or using the requests, you can send the access token like this
access_token = 'Generated_Access_token'
api_url = 'Api_Address'
# workspace id and component type
workspace_id = 1
component_type_name = "CompA"
# Define headers with the access token
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json', # Adjust content type as needed
}
# Make a GET request (you can use other HTTP methods like POST, PUT, etc.)
component_types_workspace = requests.get(api_url + "components/types/?workspace="+str(workspace_id), headers=headers)
Python API Documentation
The Python API lets you access and update objects in your deployment with python code.
Install the necessary python API package with pip:
pip install valispace
Import the API module in a python script:
import valispace
and initialize with
valispace = valispace.API()
More information about the functionalities and functions in the API can be found at here. The python API package is licensed under the MIT license, which means that anyone can contribute to the code by cloning the GitHub repository.
Endpoints
The users can access the endpoints on the rest page. To access the page, add “rest/”at the end of the API URL that can be found in the Requirements and Systems Portal und Settings and “User Tokens“. The Rest page shows the Django Swagger with all the existing endpoints.
To access the rest page, use “your_API_URL/rest/”. Leaving out the “/” at the end of the rest doesn't redirect you to the rest page. To get your API URL please go to the “User Tokens“ in the Settings.
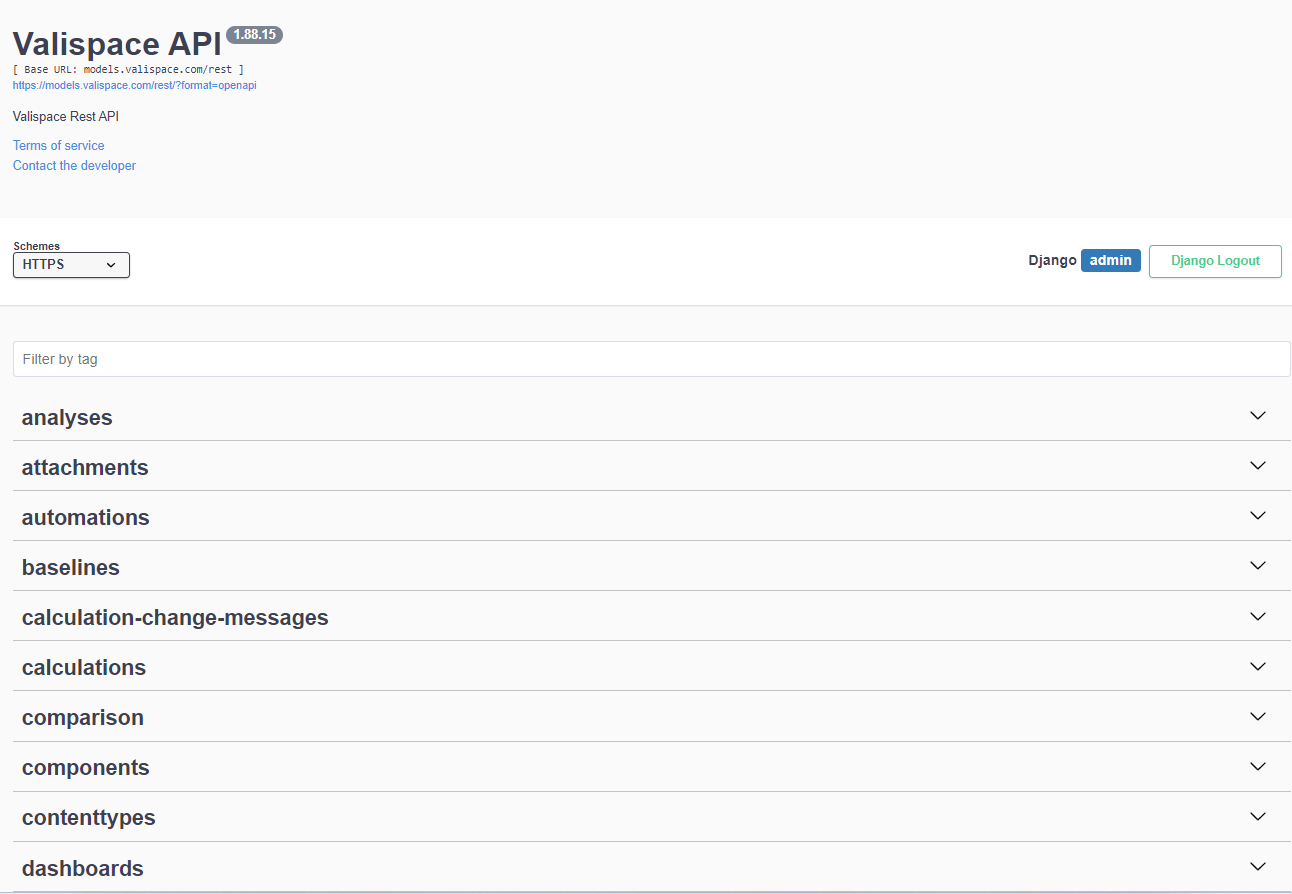
There are “GET”,” POST”,” PUT”,” PATCH” and “DELETE” methods.
Every object in the Requirements and Systems Portal has its own ID; you can use it to look for and retrieve the object information itself. The object ID can be seen either directly in the Requirements and Systems Portal or in the URL when clicking on the object in the Requirements and Systems Portal.
For example, if you use the get function to get vali information through the rest, you can use the following endpoint to get the vali details.
GET /rest/valis/{vali-id}/

Using GET, retrieving the information of the vali
Similarly, you can use the methods to retrieve/update or post different objects such as Blocks, tags, valis, textvalis, requirements, specifications, verification methods, etc. You can search for the endpoints on the rest page.
Filtering objects for projects
In addition to authentication, you can use the "GET" method to retrieve objects such as Valis or Requirements within the deployment. If you need to filter the results based on a specific project, you can achieve this by leveraging the filtering capabilities, provided the endpoint is configured accordingly. For instance, to retrieve requirements associated with project 24, you can use the following GET request:
GET /rest/requirements/?project=24
This functionality is demonstrated in the video below for a clearer understanding.
Inbuilt functions
Within the Requirements and Systems Portal, whenever a user types the text in the text field, the text is saved in the backend in the HTML
format. The HTML format retains the formatting references to valis or other objects. So, if you are getting the requirements information, you might get the HTML text in your import. To avoid this, we have implemented two functions which can be useful to convert the HTML fields to just text or HTML formatting with valis converted to text. The functions are clean_text and clean_html.
Clean_text function
The clean text function converts all the HTML formats and object references within the field to text. However, in this case, the formatting is also lost. For example, the formatting is also lost if you have a list, tables or colour. You can use this as shown below.
GET /rest/requirements/?project=24&clean_text=text,rationale
Here, I am doing the filter for project 24 and asking to give the clean text for the text and rationale of the field. The output would be this for this specific action.
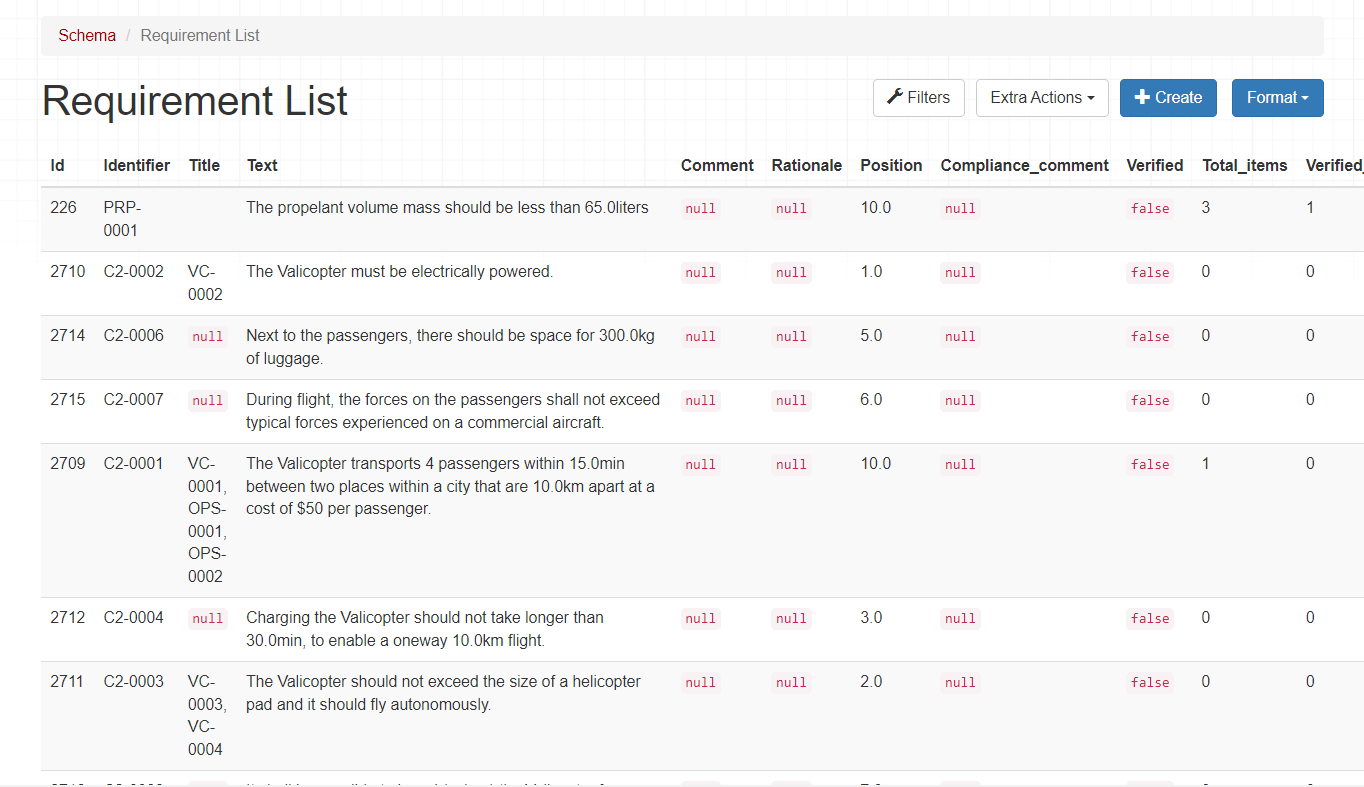
Requirements after using the Clean_text function
Clean_html function
The clean_html function keeps the formatting options of the text but then converts the references/objects like valis to text. If the text contains formatting options such as lists, background colour, tables etc, this information is retained.
GET /rest/requirements/?project=24&clean_html=text,rationale
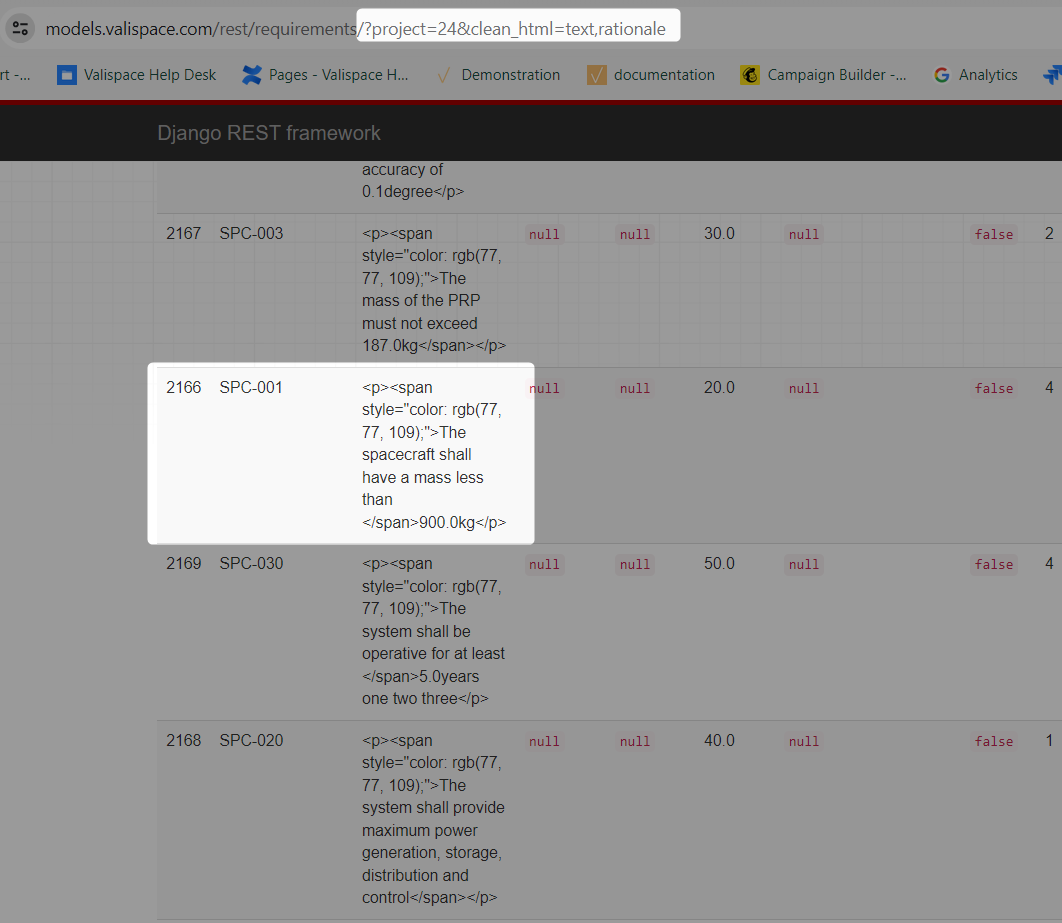
Requirements after using the clean_html function
Common Questions:
How to find the correct endpoint?
Navigating through numerous endpoints with similar names can be confusing. If you ever find uncertainty about a specific endpoint, we encourage you to contact our Altium Support Page for clarification. Alternatively, you can leverage your browser's "network" feature to observe which endpoints are triggered when performing actions within the software's front end.
As an illustration, consider the process of creating a Block. In this case, the associated endpoint can be identified as a "POST" request to "/rest/components," this information can be easily discerned by inspecting the network activity during the action. A concise demonstration of this approach is provided in the brief video below.
How do you get/post a requirement's verification methods(VM) and Blocks?
A requirement in the Requirements and Systems Portal can be associated with multiple verification methods (VMs), each of which can, in turn, have multiple Blocks attached to it. Various verification method types are supported, including rules, test, inspection, analyses, review, and custom VMs. Each verification method type is uniquely identified by its own ID.
You can use the appropriate endpoint to retrieve the IDs for these verification method types.
GET /rest/requirements/verification-methods/
For example, to obtain the IDs of Verification method types for project id 24, you can use the following endpoint
GET /rest/requirements/verification-methods/?project=24
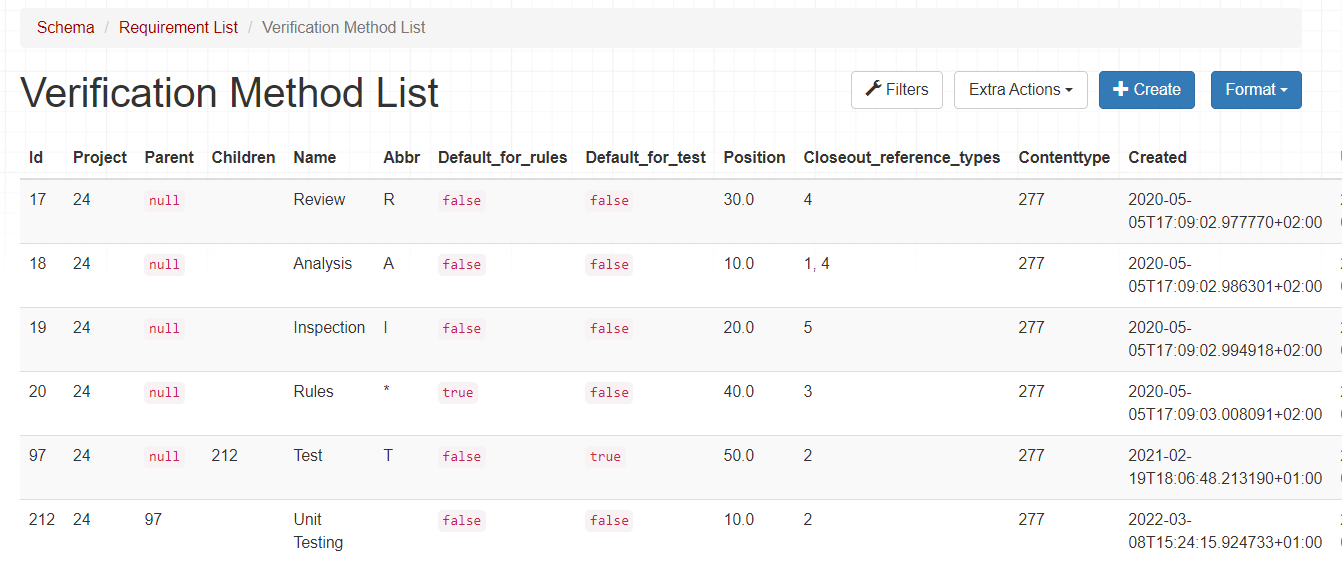
Verification method list of the project
To obtain the verification methods linked to a specific requirement, the Requirements and Systems Portal creates a dedicated object known as "Requirement-VMS." This object has its unique identifier (ID), which can be accessed through the following endpoint:
GET /rest/requirements/requirement-vms/
For example, this requirement, SPC-002 with ID 2165 has one verification method of type “Rules”, and the object id of requirment-vms is 2203.
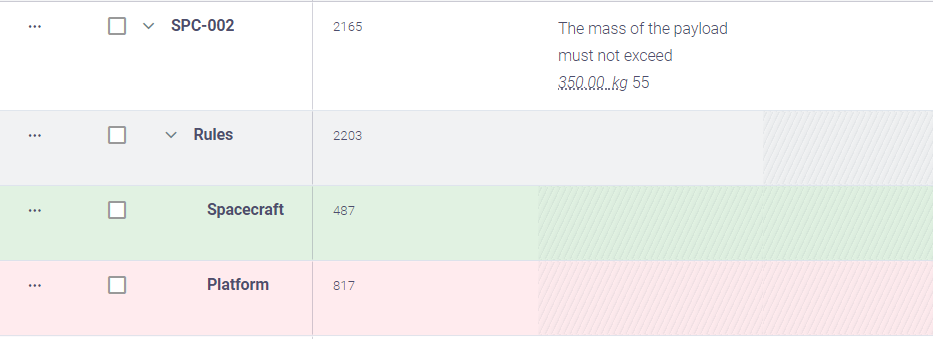
If we use the endpoint, “/rest/requirements/requirement-vms/2203
” we get the following response.
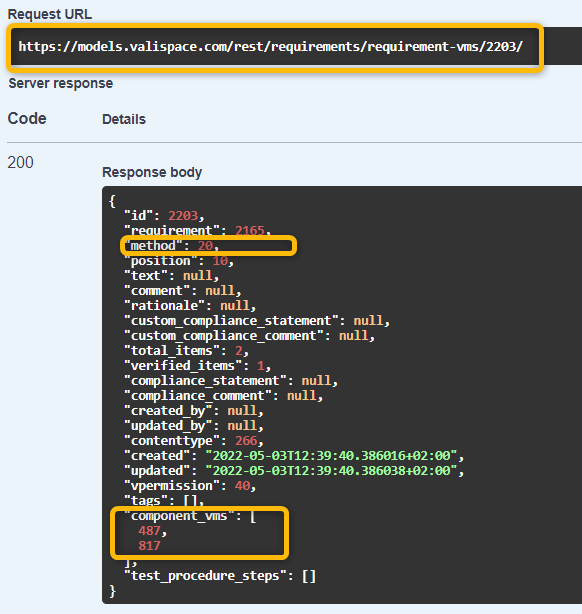
Requirment-vms keys and values
Upon inspection of the "Requirement-vms" object, you'll notice that the method ID "20" corresponds to the "Rules" Verification Method, as per the list of verification method types obtained earlier. When incorporating this information into your script, ensure that you map the method ID to the respective verification method type.
Furthermore, within the "Requirement-vms" object, there are references to two component-vms. These component-vm IDs represent the Blocks attached to the Requirement Verification Methods. When interacting with these Blocks programmatically, utilize these IDs in your script accordingly.
The endpoint to access the component-vms would be
GET /rest/requirements/component-vms/{id}
When you get the details of the component-vms, the component fields gives you the Block id with which you can map the Block’s name.
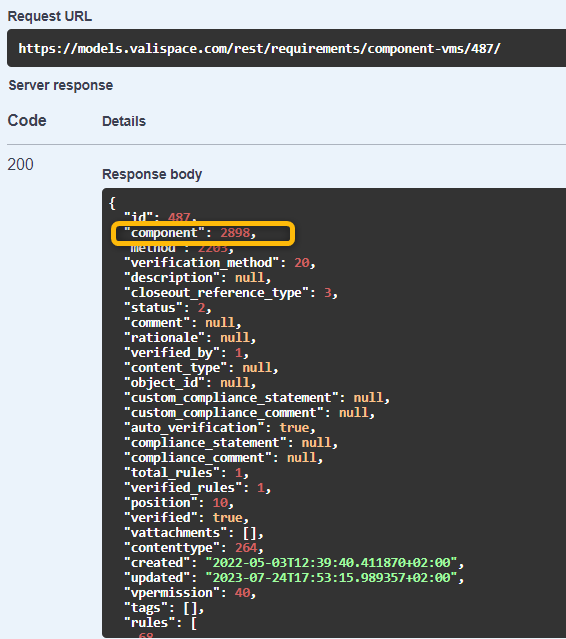
Component-vms